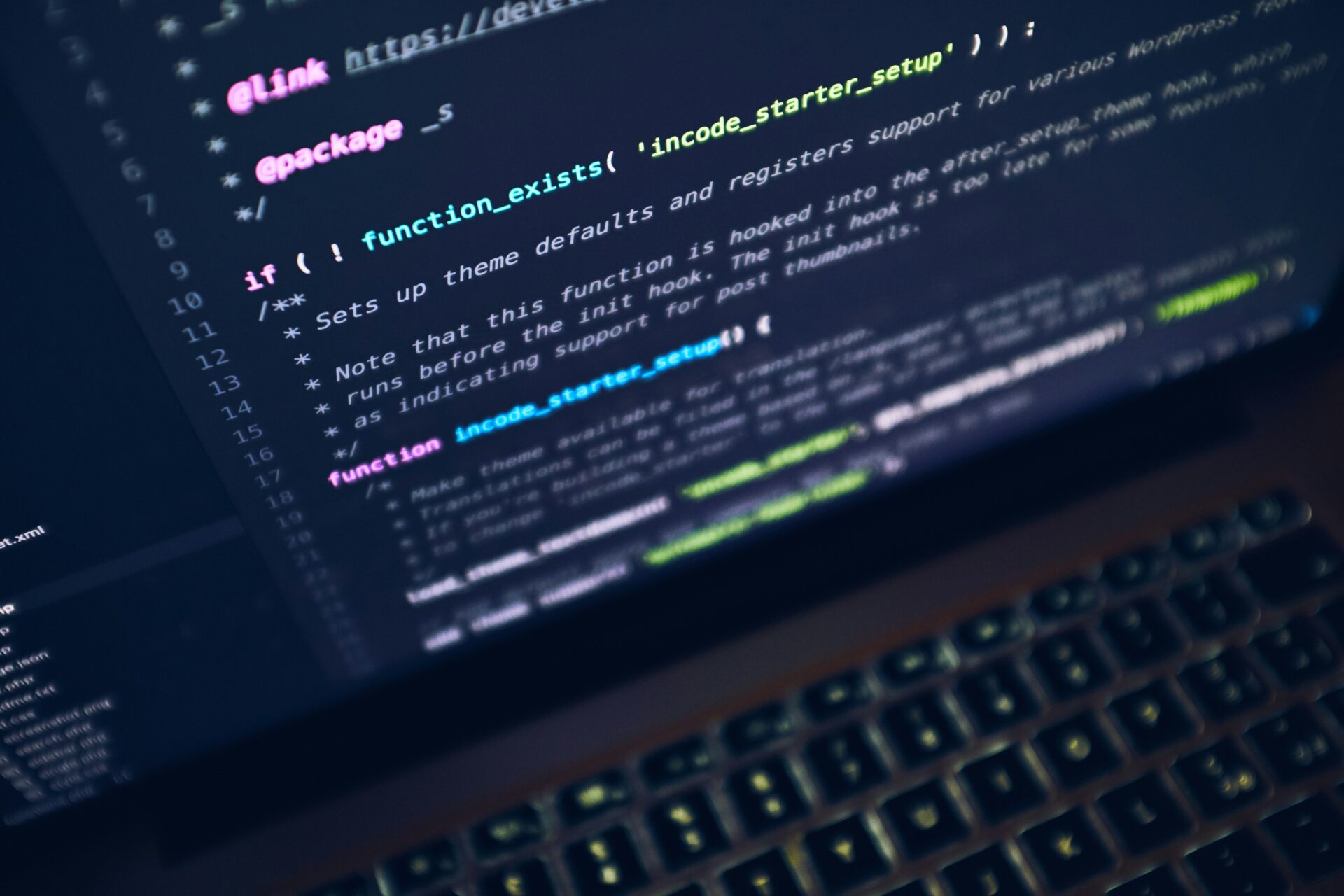
jspファイルで見られる「fn」タグの使用方法をサンプルコードを交えてご紹介します。
JSTL・Functionsタグ一覧
タグ | 説明 | 仕様 | 戻り値 |
---|---|---|---|
fn:contains | 文字列の検索 | fn:contains(‘対象文字列’, ‘検索文字’) | 第一引数の中に第二引数が存在するとtrue |
fn:containsIgnoreCase | 文字列の検索(大文字小文字区別なし) | fn:containsIgnoreCase(‘対象文字列’, ‘検索文字’) | 第一引数の中に第二引数が存在するとtrue |
fn:indexOf | 文字列の検索 | fn:indexOf(‘検索文字列’, ‘検索文字’) | 第一引数の中で第二引数の存在位置を返す |
fn:startsWith | 文字列の検証 | fn:startsWith(‘検証文字列’, ‘検証文字’) | 第一引数の始めが第二引数で始まるとtrue |
fn:endsWith | 文字列の検証 | fn:endsWith(‘検証文字列’, ‘検証文字’) | 第一引数の終わりが第二引数で始まるとtrue |
fn:trim | 文字列の前後の空白を除去 | fn:trim(‘対象文字列’) | 文字列の前後の半角空白を除去 全角空白は除去しない |
fn:length | 文字長を取得 | fn:length(‘対象文字列’) | |
fn:join | 文字配列の結合 | fn:join(‘対象配列’, ‘結合文字’) | 第一引数の配列の要素を第二引数の文字で結合する |
fn:split | 文字列を分割 | fn:split(‘対象文字列’, ‘分割文字列’) | 第一引数を第二引数で分割し、配列にする |
fn:replace | 文字列を変更 | fn:replace(‘対象文字列’, ‘変更前文字列’ , ‘変更後文字列’) | 第一引数の文字列内の第二引数の文字を第三引数に変更する |
fn:substring | 文字列の抽出 | fn:substring(‘対象文字列’, 抽出開始位置, 抽出終了位置) | 第一引数内の文字列を第二引数から第三引数の位置まで抽出する |
fn:substringAfter | 文字列の抽出 | fn:substringAfter(‘対象文字列’, ‘抽出基準文字’) | 第一引数の文字列内の第二引数の文字以降を抽出する |
fn;substringBefore | 文字列の抽出 | fn:substringBefore(‘対象文字列’, ‘抽出基準文字’) | 第一引数の文字列内の第二引数の文字以前を抽出する |
fn:escapeXml | 特殊文字列を変換 | fn:escapeXml(‘対象文字列’) | javascriptなどが実行されないようエスケープ <, > , ; などをエスケープ |
fn:toLowerCase | 文字列の小文字変換 | fn:toLowerCase(‘対象文字列’) | 全ての文字を小文字に変換する |
fn:toUpperCase | 文字列の大文字変換 | fn:toUpperCase(‘対象文字列’) | 全ての文字を大文字に変換する |
サンプルコード
環境
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.0.0</version>
<relativePath /> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>JSTLSample</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>war</packaging>
<name>JSTLSample</name>
<description>Demo project for Spring Boot</description>
<url />
<licenses>
<license />
</licenses>
<developers>
<developer />
</developers>
<scm>
<connection />
<developerConnection />
<tag />
<url />
</scm>
<properties>
<java.version>17</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-validation</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!-- JSTLの利用 -->
<dependency>
<groupId>jakarta.servlet.jsp.jstl</groupId>
<artifactId>jakarta.servlet.jsp.jstl-api</artifactId>
<version>3.0.0</version>
</dependency>
<dependency>
<groupId>org.glassfish.web</groupId>
<artifactId>jakarta.servlet.jsp.jstl</artifactId>
<version>3.0.1</version>
</dependency>
<!-- jspをコンパイル -->
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-jasper</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
application.properties
spring.application.name=JSTLSample
spring.mvc.view.prefix=/WEB-INF/views/
spring.mvc.view.suffix=.jsp
taglib.jsp
<%@ taglib uri="jakarta.tags.functions" prefix="fn"%>
ファイル階層
- /
- src/
- main/
- java
- com/example/demo/
- JstlSampleApplication.java
- ServletInitializer.java
- controller/
- FunctionsConstroller.java
- com/example/demo/
- resources/
- application.properties
- static/CSS/
- common.css
- webapp/
- WEB-INF/views/
- Functions/
- function.jsp
- taglib.jsp
- Functions/
- WEB-INF/views/
- java
- main/
- pom.xml
- …
- src/
コード
全体のfnタグをまとめたjspファイルを記載した後、タグごとに分けて記載しています。
全体像ではなく、特定のタグのサンプルが見たい方はそちらをご覧ください。
function.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ include file="./../taglib.jsp"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<c:url value="/CSS/common.css" var="stylesheet" />
<link rel="stylesheet" type="text/css" href="${stylesheet}">
<title>${title}</title>
</head>
<body>
<h1>JSTL Functions</h1>
<!-- fn:contains -->
<section>
<h2>fn:contains</h2>
<div class="code-block">
<c:set var="containsKey" value="containsTest" />
<c:if test="${fn:contains(containsKey, 'contain')}">
true
</c:if>
<c:if test="${!fn:contains(containsKey, 'contain')}">
false
</c:if>
<c:if test="${fn:contains(containsKey, 'CONTAIN')}">
CONTAIN true
</c:if>
</div>
<h2>fn:containsIgnoreCase</h2>
<div class="code-block">
<c:set var="ignoreCaseKey" value="ignoreCaseTest" />
<c:if test="${fn:containsIgnoreCase(ignoreCaseKey, 'IGNORE')}">
true
</c:if>
<c:if test="${!fn:containsIgnoreCase(ignoreCaseKey, 'IGNORE')}">
false
</c:if>
</div>
<h2>fn:indexOf</h2>
<div class="code-block">
<c:set var="indexKey" value="indexValue" />
<c:out value="${fn:indexOf(indexKey, 'Value')}" />
</div>
<h2>fn:startsWith</h2>
<div class="code-block">
<c:set var="startKey" value="startTest" />
<c:if test="${fn:startsWith(startKey, 'Start')}">
true
</c:if>
<c:if test="${!fn:startsWith(startKey, 'Start')}">
false
</c:if>
</div>
<h2>fn:endsWith</h2>
<div class="code-block">
<c:set var="endKey" value="endTest" />
<c:if test="${fn:endsWith(endKey, 'Test')}">
true
</c:if>
<c:if test="${!fn:endsWith(endKey, 'Test')}">
false
</c:if>
</div>
<h2>fn:trim</h2>
<div class="code-block">
<c:set var="trimKey" value=" trim " />
<c:out value='\"${fn:trim(trimKey)}\"' />
</div>
<h2>fn:length</h2>
<div class="code-block">
<c:set var="lengthKey" value="length" />
<c:out value="${fn:length(lengthKey)}" />
</div>
<h2>fn:join</h2>
<div class="code-block">
<%
String[] joinArray = new String[3];
joinArray[0] = "join1";
joinArray[1] = "join2";
joinArray[2] = "join3";
pageContext.setAttribute("joinArray", joinArray);
%>
<c:out value="${fn:join(joinArray, ', ')}" />
</div>
<h2>fn:split</h2>
<div class="code-block">
<c:set var="splitKey" value="split1,split2,split3" />
<c:forEach var="split" items="${fn:split(splitKey,',')}">
${split}
</c:forEach>
</div>
<h2>fn:replace</h2>
<div class="code-block">
<c:set var="replaceKey" value="replace" />
<c:out value="${fn:replace(replaceKey, 'replace', 'REPLACE')}" />
</div>
<h2>fn:substring</h2>
<div class="code-block">
<c:set var="substringKey" value="SuBStRinG" />
<c:out value="${fn:substring(substringKey, 0, 6)}" />
</div>
<h2>fn:substringAfter</h2>
<div class="code-block">
<c:set var="After" value="substringAFTER" />
<c:out value="${fn:substringAfter(After, 'substring')}" />
</div>
<h2>fn:substringBefore</h2>
<div class="code-block">
<c:set var="before" value="substringBEFORE" />
<c:out value="${fn:substringBefore(before, 'BEFORE')}" />
</div>
<h2>fn:escapeXml</h2>
<div class="code-block">
<c:set var="escapeKey" value="<big>escape</big>" />
nomal : ${escapeKey}<br> escapeXml : ${fn:escapeXml(escapeKey)}
</div>
<h2>fn:toLowerCase</h2>
<div class="code-block">
<c:set var="lower" value="LOWercAse" />
<c:out value="${fn:toLowerCase(lower)}" />
</div>
<h2>fn:toUpperCase</h2>
<div class="code-block">
<c:set var="upper" value="toUPperCaSE" />
<c:out value="${fn:toUpperCase(upper)}" />
</div>
</section>
</body>
</html>
タグごと
fn:contains
<h2>fn:contains</h2>
<div class="code-block">
<c:set var="containsKey" value="containsTest" />
<c:if test="${fn:contains(containsKey, 'contain')}">
true
</c:if>
<c:if test="${!fn:contains(containsKey, 'contain')}">
false
</c:if>
<c:if test="${fn:contains(containsKey, 'CONTAIN')}">
CONTAIN true
</c:if>
</div>
fn:containsIgnoreCase
<h2>fn:containsIgnoreCase</h2>
<div class="code-block">
<c:set var="ignoreCaseKey" value="ignoreCaseTest" />
<c:if test="${fn:containsIgnoreCase(ignoreCaseKey, 'IGNORE')}">
true
</c:if>
<c:if test="${!fn:containsIgnoreCase(ignoreCaseKey, 'IGNORE')}">
false
</c:if>
</div>
fn:indexOf
<h2>fn:indexOf</h2>
<div class="code-block">
<c:set var="indexKey" value="indexValue" />
<c:out value="${fn:indexOf(indexKey, 'Value')}" />
</div>
fn:startsWith
<h2>fn:startsWith</h2>
<div class="code-block">
<c:set var="startKey" value="startTest" />
<c:if test="${fn:startsWith(startKey, 'Start')}">
true
</c:if>
<c:if test="${!fn:startsWith(startKey, 'Start')}">
false
</c:if>
</div>
fn:endsWith
<h2>fn:endsWith</h2>
<div class="code-block">
<c:set var="endKey" value="endTest" />
<c:if test="${fn:endsWith(endKey, 'Test')}">
true
</c:if>
<c:if test="${!fn:endsWith(endKey, 'Test')}">
false
</c:if>
</div>
fn:trim
<h2>fn:trim</h2>
<div class="code-block">
<c:set var="trimKey" value=" trim " />
<c:out value='\"${fn:trim(trimKey)}\"' />
</div>
fn:length
<h2>fn:length</h2>
<div class="code-block">
<c:set var="lengthKey" value="length" />
<c:out value="${fn:length(lengthKey)}" />
</div>
fn:join
<h2>fn:join</h2>
<div class="code-block">
<%
String[] joinArray = new String[3];
joinArray[0] = "join1";
joinArray[1] = "join2";
joinArray[2] = "join3";
pageContext.setAttribute("joinArray", joinArray);
%>
<c:out value="${fn:join(joinArray, ', ')}" />
</div>
fn:split
<h2>fn:split</h2>
<div class="code-block">
<c:set var="splitKey" value="split1,split2,split3" />
<c:forEach var="split" items="${fn:split(splitKey,',')}">
${split}
</c:forEach>
</div>
fn:replace
<h2>fn:replace</h2>
<div class="code-block">
<c:set var="replaceKey" value="replace" />
<c:out value="${fn:replace(replaceKey, 'replace', 'REPLACE')}" />
</div>
fn:substring
<h2>fn:substring</h2>
<div class="code-block">
<c:set var="substringKey" value="SuBStRinG" />
<c:out value="${fn:substring(substringKey, 0, 6)}" />
</div>
fn:substringAfter
<h2>fn:substringAfter</h2>
<div class="code-block">
<c:set var="After" value="substringAFTER" />
<c:out value="${fn:substringAfter(After, 'substring')}" />
</div>
fn:substringBefore
<h2>fn:substringBefore</h2>
<div class="code-block">
<c:set var="before" value="substringBEFORE" />
<c:out value="${fn:substringBefore(before, 'BEFORE')}" />
</div>
fn:escapeXml
<h2>fn:toLowerCase</h2>
<div class="code-block">
<c:set var="lower" value="LOWercAse" />
<c:out value="${fn:toLowerCase(lower)}" />
</div>
fn:toLowerCase
<h2>fn:toLowerCase</h2>
<div class="code-block">
<c:set var="lower" value="LOWercAse" />
<c:out value="${fn:toLowerCase(lower)}" />
</div>
fn:toUpperCase
<h2>fn:toUpperCase</h2>
<div class="code-block">
<c:set var="upper" value="toUPperCaSE" />
<c:out value="${fn:toUpperCase(upper)}" />
</div>
画面表示
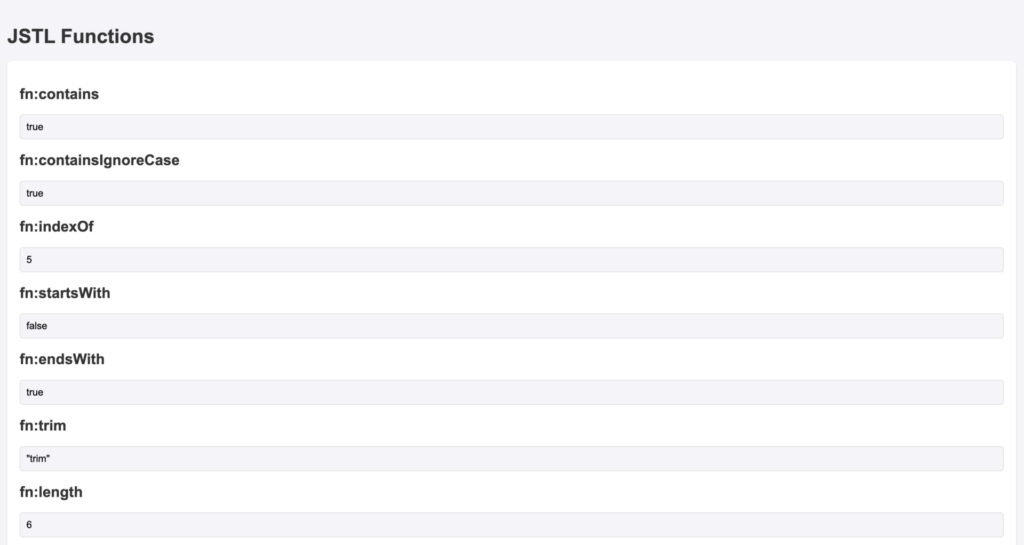
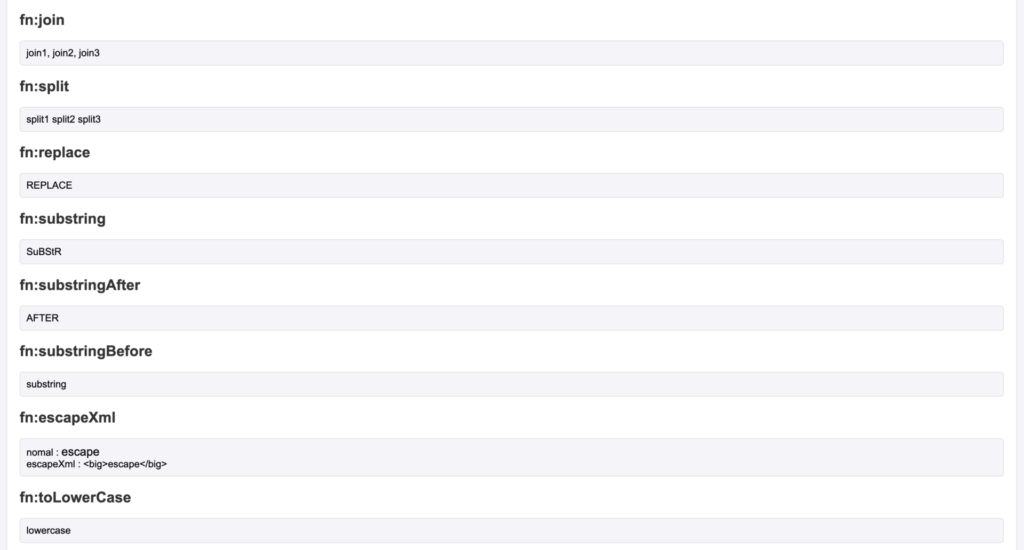

サンプルコード詳細
環境
pom.xml
jsp、JSTLを使用するにあたって必須の依存関係があります。
<!-- JSTLの利用 -->
<dependency>
<groupId>jakarta.servlet.jsp.jstl</groupId>
<artifactId>jakarta.servlet.jsp.jstl-api</artifactId>
<version>3.0.0</version>
</dependency>
<dependency>
<groupId>org.glassfish.web</groupId>
<artifactId>jakarta.servlet.jsp.jstl</artifactId>
<version>3.0.1</version>
</dependency>
<!-- jspをコンパイル -->
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-jasper</artifactId>
</dependency>
上記の依存関係は必須です。
2〜11行目がJSTLの使用を可能にし、
13=16行目がjspの使用を可能にしています。
また、jspを表示する際に、必須の設定がapplication.propertiesにもあります。
application.properties
spring.mvc.view.prefix=/WEB-INF/views/
spring.mvc.view.suffix=.jsp
上記の2行、特に2行目の設定は必須です。(1行目は任意)
2行目の設定がなければ、HTMLファイルを行事しようとするため、
jspファイルの表示がされません。(jspファイルを表示せず、ダウンロードしてしまう)
そのため、拡張子をデフォルトの.htmlから.jspへ変えているのが2行目です。
1行目は任意です。jspファイルのルートディレクトリを設定しています。
コード(タグごと)
fn:contains
<h2>fn:contains</h2>
<div class="code-block">
<c:set var="containsKey" value="containsTest" />
<c:if test="${fn:contains(containsKey, 'contain')}">
true
</c:if>
<c:if test="${!fn:contains(containsKey, 'contain')}">
false
</c:if>
<c:if test="${fn:contains(containsKey, 'CONTAIN')}">
CONTAIN true
</c:if>
</div>
文字列のチェックをします。
3行目で「containsKey」という名前の変数をセットしています。
値は「containsTest」です。
5行目以降で変数の値をチェックしています。
具体的には、この変数内に「contain」という値が含まれているかをチェックしています。
含まれていた場合はtrueを返し、c:ifタグ内を表示します。
今回のサンプルではtrueになるため、6行目のtrueという文字が画面に表示されています。
fn:containsIgnoreCase
<h2>fn:containsIgnoreCase</h2>
<div class="code-block">
<c:set var="ignoreCaseKey" value="ignoreCaseTest" />
<c:if test="${fn:containsIgnoreCase(ignoreCaseKey, 'IGNORE')}">
true
</c:if>
<c:if test="${!fn:containsIgnoreCase(ignoreCaseKey, 'IGNORE')}">
false
</c:if>
</div>
文字列のチェックを大文字、小文字の区別をせずにします。
3行目で「ignoreCaseKey」という名前の変数をセットしています。
値は「ignoreCaseTest」です。
5行目以降で変数の値をチェックしています。
具体的には、大文字小文字の区別をせず、
変数内に「IGNORE」という文字が含まれているかをチェックしています。
サンプルでは、変数内に「ignore」という小文字が含まれているため、
trueとなり、c:ifタグ内のtrueという文字を表示しています。
fn:indexOf
<h2>fn:indexOf</h2>
<div class="code-block">
<c:set var="indexKey" value="indexValue" />
<c:out value="${fn:indexOf(indexKey, 'Value')}" />
</div>
文字列の位置を取得します。
3行目で、「indexKey」という名前の変数をセットしています。
値は「indexValue」です。
4行目でfn:indexOfを使用し、取得した値を出力しています。
具体的には、「Value」という文字が「indexKey」の値「indexValue」内の
何番目に位置するかを出力しています。
サンプルの場合、
値 | i | n | d | e | x | V | a | l | u | e |
番号 | 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 |
となっているため、「5」が画面に出力されています。
fn:startsWith
<h2>fn:startsWith</h2>
<div class="code-block">
<c:set var="startKey" value="startTest" />
<c:if test="${fn:startsWith(startKey, 'Start')}">
true
</c:if>
<c:if test="${!fn:startsWith(startKey, 'Start')}">
false
</c:if>
</div>
文字列の始まりをチェックします。
3行目で「startKey」という変数に「startTest」という値をセットしています。
この変数を5行目以降のstartsWtihでチェックしています。
具体的には、「startKey」の値が「Start」という文字で始まるかをチェックしています。
サンプルでは、「start」で始まり、「Start」と頭文字が大文字ではないため、
falseとなり、10行目の「false」が画面出力だれています。
fn:endsWith
<h2>fn:endsWith</h2>
<div class="code-block">
<c:set var="endKey" value="endTest" />
<c:if test="${fn:endsWith(endKey, 'Test')}">
true
</c:if>
<c:if test="${!fn:endsWith(endKey, 'Test')}">
false
</c:if>
</div>
文字列の終わりをチェックします。
3行目で「endKey」という変数名に「endTest」という値をセットしています。
それを5行目以降のendsWithでチェックしています。
具体的には、「endKey」の値が「Test」という文字で終わるかをチェックしています。
サンプルでは「Test」で終わっているため、trueとなり、
6行目のtrueが画面表示されています。
fn:trim
<h2>fn:trim</h2>
<div class="code-block">
<c:set var="trimKey" value=" trim " />
<c:out value='\"${fn:trim(trimKey)}\"' />
</div>
文字列の前後の半角空白を除去します。
3行目で、「trimKey」という変数に、「 trim 」という値をセットしています。
ここではあえて前後に空白付きでセットしています。
この空白付きの変数を、4行目のfn:trimで削除し、ダブルクォーテーション付きで出力してます。
画面表示は、変数の前後の半角空白が除去され、「trim」と出力されます。
fn:length
<h2>fn:length</h2>
<div class="code-block">
<c:set var="lengthKey" value="length" />
<c:out value="${fn:length(lengthKey)}" />
</div>
文字長を取得します。
3行目で「lengthKey」という変数に「length」という値をセットしています。
この文字長を4行目のfn:lengthにて取得しています。
「length」は6文字のため、画面には「6」と出力されます。
fn:join
<h2>fn:join</h2>
<div class="code-block">
<%
String[] joinArray = new String[3];
joinArray[0] = "join1";
joinArray[1] = "join2";
joinArray[2] = "join3";
pageContext.setAttribute("joinArray", joinArray);
%>
<c:out value="${fn:join(joinArray, ', ')}" />
</div>
配列を指定文字で結合します。
4~8行目で配列をセットしています。
それを10行目で出力しています。
出力の際に、配列を半角カンマと半角空白(, )で結合しているので、
元の配列が結合され、
join1, join2, join3
と画面表示されています。
fn:split
<h2>fn:split</h2>
<div class="code-block">
<c:set var="splitKey" value="split1,split2,split3" />
<c:forEach var="split" items="${fn:split(splitKey,',')}">
${split}
</c:forEach>
</div>
文字列を指定文字で分割し、配列にします。
3行目でカンマ区切りの文字列をセットしています。
それを4行目のfn:splitで半角カンマで分割し、
[split1, split2, split3]
という配列にしています。
これをc:forEachでループさせているため、
5行目でこの配列の値が出力されます。
fn:replace
<h2>fn:replace</h2>
<div class="code-block">
<c:set var="replaceKey" value="replace" />
<c:out value="${fn:replace(replaceKey, 'replace', 'REPLACE')}" />
</div>
文字列の置換をします。
3行目で「replaceKey」という変数に「replace」という値をセットしています。
この値を4行目のfn:replaceで置換しています。
具体的には、fn:replaceで、変数replaceKey内の「replace」を「REPLACE」へ
置換しています。
今回のサンプルの例では、変数replaceKeyのすべての値が「REPLACE」へと
置換されるため、画面には「REPLACE」と出力されています。
fn:substring
<h2>fn:substring</h2>
<div class="code-block">
<c:set var="substringKey" value="SuBStRinG" />
<c:out value="${fn:substring(substringKey, 0, 6)}" />
</div>
文字列の抽出をします。
3行目で「substringKey」という変数に「SuBStRinG」という値をセットしています。
この変数を4行目のfn:substringで抽出して表示しています。
具体的には、sybstringKeyの値の先頭(0)から6文字分を抽出しています。
値 | S | u | B | S | t | R | i | n | G |
番号 | 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 |
上記の図より、始め(0)から6文字抽出し、「SuBStR」が画面表示されています。
fn:substringAfter
<h2>fn:substringAfter</h2>
<div class="code-block">
<c:set var="After" value="substringAFTER" />
<c:out value="${fn:substringAfter(After, 'substring')}" />
</div>
指定文字以降の文字を抽出します。
3行目で「After」という変数に「substringAFTER」という値をセットしています。
これを元に4行目のfn:substringAfterで抽出しています。
具体的には、「substrngAFTER」の「substring」以降を抽出するように指定しているので、
「AFTER」が画面表示されます。
fn:substringBefore
<h2>fn:substringBefore</h2>
<div class="code-block">
<c:set var="before" value="substringBEFORE" />
<c:out value="${fn:substringBefore(before, 'BEFORE')}" />
</div>
指定文字以前の文字を抽出します。
3行目で「before」という変数に「substringBEFORE」という値をセットしてます。
これを元に、4行目のfn:substringBeforeで抽出しています。
具体的には、「substringBEFORE」の「BEFORE」以前を抽出するよう指定しているので、
「substring」が画面表示されます。
fn:escapeXml
<h2>fn:escapeXml</h2>
<div class="code-block">
<c:set var="escapeKey" value="<big>escape</big>" />
nomal : ${escapeKey}<br> escapeXml : ${fn:escapeXml(escapeKey)}
</div>
文字列のエスケープをします。
クロスサイトスクリプティング(XSS)などの対策です。
3行目で「escapeKey」という変数に、タグ付きの値、「<big>escape</big>」をセットしています。
この変数を4行目にて2パターンで出力しています。
前半ではそのまま表示し、後半ではfn:escapeXmlを用いてエスケープして出力しています。
そのため、前半は大きな文字で「escape」と画面表示され、
後半は、そのまま「<big>escape</big>と画面表示されています。
fn:toLowerCase
<h2>fn:toLowerCase</h2>
<div class="code-block">
<c:set var="lower" value="LOWercAse" />
<c:out value="${fn:toLowerCase(lower)}" />
</div>
文字列を全て小文字に変換します。
3行目で「lower」という変数に「LOWercAse」という大文字小文字が入り混ざった値をセットしています。
これを4行目で、fn:toLowerCaseを用いて出力しているため、
「lowercase」と全て小文字で画面表示されます。
fn:toUpperCase
<h2>fn:toUpperCase</h2>
<div class="code-block">
<c:set var="upper" value="toUPperCaSE" />
<c:out value="${fn:toUpperCase(upper)}" />
</div>
全ての小文字を大文字に変換します。
3行目で「upper」という変数に「toUPperCaSE」という大文字小文字が入り混ざった値をセットしています。
これを4行目でfn:toUppseCaseを用いて出力しているため、
「TOYPPERCASE」と全て大文字で画面出力されています。
参考サイト